Get the Arguments Under Control - Running HyperMesh 2025 in Batch with Python


Executing a Python automation script in batch mode can be very powerful. You can perform fully automated tasks without any user interaction, thus enabling you to incorporate such tasks into bigger, more complex processes.
Although the user is not expected to interact with the process, it is still desirable to offer some level of initial control. In other words, as the author of the Python script, you might want to expose certain parameters externally and turn them into command line arguments. That way not every aspect of the automation is hard-coded, and your solution becomes much more flexible and versatile.
How to Tame the Arguments in Batch Mode
Let us work with a hypothetical python_script.py that takes three arguments: arg1 (string), arg2 (float), and arg3 (integer). When you run the HyperMesh application in batch mode together with such Python script, the submission line in the command console gets a bit… Well, it looks quite busy:
"C:\Program Files\Altair\2025\hwdesktop\hwx\bin\win64\runhwx.exe" -client HyperWorksDesktop -plugin HyperworksLauncher -profile HyperworksLauncher -l en -startwith HyperMesh -f "C:\WORK\Projects\PythonAPI\python_script.py" -arg1 stringvalue -arg2 3.5 -arg3 57
There is a series of arguments required by the application itself, followed by the Python script (the -f
option), which is then followed by your script arguments. What does the Python interpreter make of all of this?
(In the examples below, we ran the HyperMesh session interactively so we could more easily observe the argument parsing in the Python console. For a batch execution without the GUI, you will simply have to add -b
to the list of application arguments.)
As you can see in the screenshot below, in HyperMesh 2025 sys.argv
will give you access to all arguments. However, all of them are treated as strings and on top of that, your custom arguments are "sandwiched" between the application arguments (see -platform
at the end of the list).
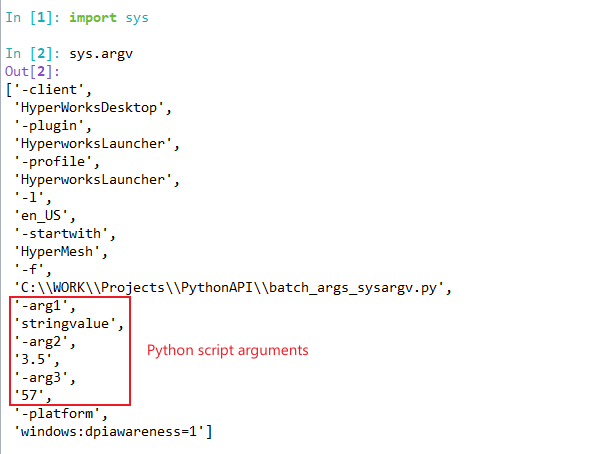
There are multiple ways to parse your arguments, working with the content of sys.argv
being one of them. If you are passing multiple arguments to your script, possibly of different data type as well, a much more elegant solution can be easily set up using the argparse
module.
Let us go back to our three arguments (string, float, and integer). As the owner of the code, you know their names and types. Hence, at the start of your code you can set up a parser
object that "understands" your arguments:
import argparse
parser = argparse.ArgumentParser()
parser.add_argument('-arg1',type=str)
parser.add_argument('-arg2',type=float)
parser.add_argument('-arg3',type=int)
args, unknown = parser.parse_known_args()
print("arg1", type(args.arg1), args.arg1)
print("arg2", type(args.arg2), args.arg2)
print("arg3", type(args.arg3), args.arg3)
What does the above code do? Firstly, we created the parser object. Secondly, we told the parser which arguments we are expecting by specifying their respective name and type. Finally, we called the parse_known_args
method on the parser object to give us a two-item tuple containing the populated namespace and the list of "unknown" argument strings.
The first object in the tuple contains all the known arguments represented by the desired data type.
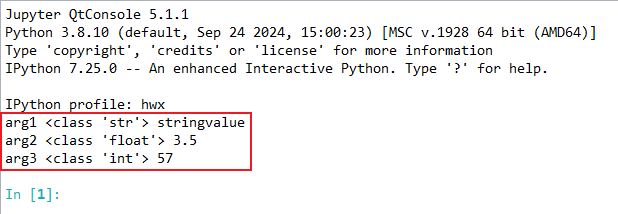
You can find more details about the argparse module in the official Python documentation (see link below) or a quick web search will lead you to many nice articles/blogs covering this topic.
argparse — Parser for command-line options, arguments and subcommands — Python 3.13.1 documentation
Enjoy the batch mode with Python!
Hi!
I tried with -b in the application arguments but nothing is showing up.
Can you provide an example with which where you are supposed to place -b? I wrote my snippet of command prompt code like this:
f'runhwx.exe -client HyperWorksDesktop -b -plugin HyperworksLauncher -profile HyperworksLauncher -l en -startwith HyperMesh -f "{hmTempFilePath}"'