Post-process CAE data and visualize in HyperView automatically using Altair Compose
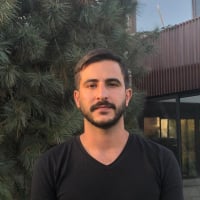

Automating your CAE workflows is often as important as creating them in the first place. You can use Altair Compose to turn a complex CAE workflow that requires heavy user interaction into an efficient process requiring a single click. In this short article I show how to automate a simple workflow that does the following:
- Read H3D file.
- Post-process data to calculate new custom results.
- Write custom results in a new H3D.
- Write TCL script for displaying model & results in HyperView.
- Launch HyperView and run created TCL script.
Let’s go through the necessary steps to do this in Compose. First, the data to be read from the H3D input file is defined. Specifically: file name, subcase to read from, datatype to read (elemental stresses) and component (von Mises stress). The list of element IDs is read from the model because, afterwards, it is required to write post-processed data into its corresponding element.
close all, clear, clc
% Define input file & data to read from it
inputFile = 'Sub-Wall-Cutout.h3d';
subcase = 'Subcase 1 (pressure load)';
datatype = 'Element Stresses (2D & 3D) (2D)';
component = 'vonMises (Z1)';
% Get model's element list for writing new data into H3D file
requestsCell = getreqlist(inputFile, subcase, datatype);
requests = cellfun(@(req) str2num(req(2:end)), requestsCell);
Next step is reading von Mises stress data from the file. The readcae command takes the file name, subcase, datatype and component defined previously. It also requires the requests (elements or nodes of interest) and the timesteps to read from. Setting these inputs as square brackets [ ] means extracting all elements and all timesteps (this file contains a single timestep, though).
The last input for this command can be either 0 or 1. It defines if the read data will be returned as a cell (0) or as a matrix (1). The next lines just calculate a safety factor for each element as compared to the maximum stress present in the model (just for illustrative purposes).
% Read von Mises stress data from input file & calculate safety factor
vm = readcae(inputFile, subcase, datatype, [], component, [], 1);
vmMax = max(vm);
SF = vmMax ./ vm;
Afterwards, the data can be read in a straightforward process:
- A new H3D file is created.
- A datatype and a subcase are defined inside it.
- Data is written into the file.
- File is closed.
There are a few details to specify during this process. Namely:
- createh3ddatatype: Datatype is called Safety Factor and defined as a scalar value, bound to elements rather than nodes.
- createh3dsubcase: Subcase is called Subcase 1 and a single timestep (0) will be stored in it.
- writeh3ddata: Zeros given as inputs indicate data won’t be associated to any layer and no corner data will be written.
% Write safety factor data into new H3D file
outputFile = 'Sub-Wall-Cutout - SF.h3d';
fid = createh3dfile(outputFile);
dtName = 'Safety Factor';
dt = createh3ddatatype(fid, dtName, 'scalar', 'elem');
sc = createh3dsubcase(fid, 'Subcase 1', 0);
R = writeh3ddata(fid, sc, 1, dt, 0, 0, requests, SF);
closeh3dfile(fid);
Once the H3D file with new results is ready, a TCL file must be created. The file will contain a few instructions to display the newly created results in the original file’s model. A TCL file is created and written into using the printf conventions of C language. These commands:
% Create .tcl script to visualize SF data alongside model in HyperView
tclFile = 'C:\Users\rsanchez\Desktop\Visualization.tcl';
fid = fopen(tclFile, 'w');
fprintf(fid, 'hwc config resultmathtemplate None\n');
fprintf(fid, 'hwc open animation model {%s}\n', fullfile(pwd, inputFile));
fprintf(fid, 'hwc open animation result {%s}\n', fullfile(pwd, outputFile));
fprintf(fid, 'hwc result scalar load type = "%s" system = global\n', dtName);
fprintf(fid, 'hwc show legends');
fclose(fid);
Will create a TCL file like this:
hwc config resultmathtemplate None
hwc open animation model {C:\Users\rsanchez\Documents\Community\Knowledge Base\Post-process CAE data and visualize in HyperView automatically using Altair Compose\Sub-Wall-Cutout.h3d}
hwc open animation result {C:\Users\rsanchez\Documents\Community\Knowledge Base\Post-process CAE data and visualize in HyperView automatically using Altair Compose\Sub-Wall-Cutout - SF.h3d}
hwc result scalar load type = "Safety Factor" system = global
hwc show legends
Note: I’m using a path with no spaces as these can lead to unexpected behavior when calling HyperView in batch.
Finally, after defining the path to the Altair installation to be used (no need to do this if Compose and HyperMesh are present in the same directory), the AltairIntegration OML library is leveraged to launch HyperView and run the TCL script.
% Call HyperView in batch to run created .tcl script
altairFolder = 'C:\Program Files\Altair\2023';
setaltairfolder(altairFolder);
callaltairbatch('HyperView', '-tcl', tclFile);
HyperView will launch and load the input file’s model alongside the custom results in a contour:
I hope you can take this simple example to the next level and automate more complex workflows! All files needed to replicate this are attached. Remember you can always reach out to a community of experts in the Compose Community Forum to discuss and get advice.